Heyas! I do not have a TEK2 controller but I do have Grids with endless encoders. The code may or may not be the same as I can’t see what ‘Tabs’ you have in the editor and I can’t find documentation for the endless_direction() function. The info @ docs.intech.studio likely hasn’t been updated yet.
Just to preface things, I am new with the Grid controllers and I, personally, find it a bit easier to work with straight LUA. I’m new to LUA and coding in general but it’s been pretty easy to pickup.
If you want to see what a particular action block is doing, you can check its box and click the ‘[…]’ ‘Merge to code’ button. I usually start with action blocks then convert all to code and make one monolithic ‘Code’ action block.
To achieve this using an endless encoder:
On the ‘Init’ tab for the encoder, add the ‘EC’ action to set the encoder mode. Set it to ‘1’ for ‘Relative BinOffset’:
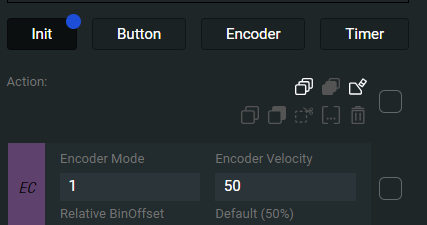
Next, on the ‘Encoder’ tab (this might be labelled different as the jogwheel on the TEK2 is a new element in the Grid world), I use the following code in place of all action blocks:
local num, val = self:element_index(), self:encoder_value()
if val == 63 then
keyboard_send(25, 0, 2, 99)
elseif val == 65 then
keyboard_send(25, 0, 2, 54)
end;
When using EC mode 1 for Relative BinOffset, the value of self:encoder_value() is 63 when turning left and 65 when turning right (I believe it returns to a value of 64 when not being turned as well). So what the code above is saying is:
IF ‘val’ (which is the value returned from self:encoder_value() which is determined by the direction you’re turning) is equal to 63 then send out the following keyboard command. Or ELSE IF ‘val’ is equal to 65 then send out the following keyboard command. Otherwise, do nothing at all.
The parameters for keyboard_send() are set when you use a ‘Keyboard’ action block:
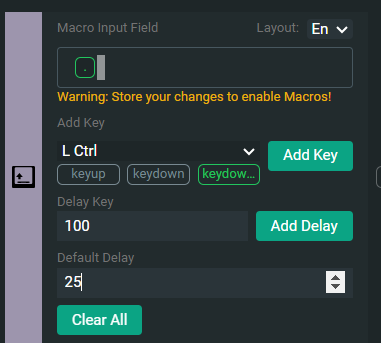
Again, I’m not sure it the jogwheel on the TEK2 is a different element than an encoder (they’re fundamentally the same), so you may need functions that address the jogwheel specifically.
My code above works to send out ‘.’ on left turn and ‘,’ on right turn (tested in Notepad on Windows). I think those are in reverse of what you need.
Let me know if that points you in the right direction. If the jogwheel does have specific functions to address its values/behaviors then, the devs will need to jump in. The docs don’t appear to be updated yet.